SMTP & IMAP Using SSL in Java Android
I. IMAP & IMAPS (IMAP using SSL):
1 – IMAP: Internet Access Message Protocol – is an Application Layer Internet protocol that allows an e-mail client to access e-mail on a remote mail server.
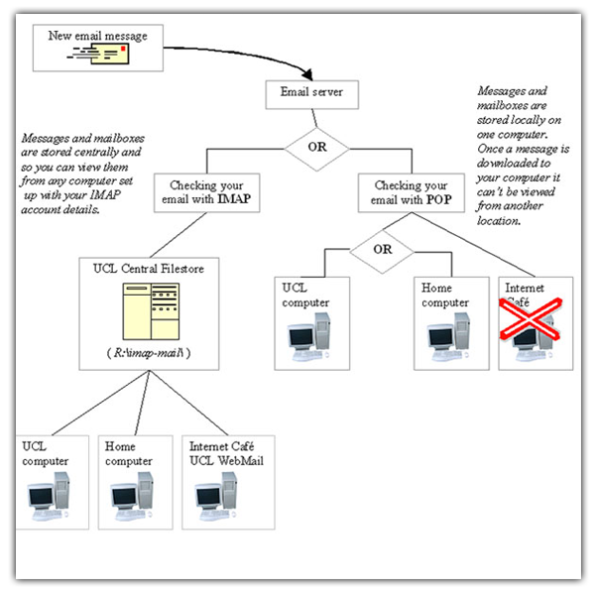
POP & IMAP Working Flow
2 – IMAPS – IMAP using SSL.
SSL: Secure Sockets Layer, is a general-purpose or multi-purpose protocol which designed to create communication between two application programs on a specified port (socket 443) to encrypt all sent and received information. Nowaday it is used for electronic information transactions such as transfer credit card numbers, passwords, secret personal number (PIN) on the Internet.
SSL was designed and first developed in 1994 by the research team lead by Netscape & Elgammal and today has become the standard security practices on the Internet. SSL version 3.0 is still being edited and improved. The below image is a sample “How do SSL work”.
The algorithm of encryption and authentication using SSL is included (version 3.0):
DES – Data encryption standard (born 1977), invented and used by the U.S. government
DSA – digital signature algorithm, standard electronic identity, invented and used by the U.S. government
Planning – key exchange algorithm, invented and used by the U.S. government
MD5 – algorithms create value “hash (message digest), invented by Rivest;
RC2, RC4 – Rivest encryption, developed by RSA Data Security Company;
RSA – public key algorithm for encryption and authentication, developed by Rivest, Shamir and Adleman;
RSA key exchange – key exchange algorithm for SSL based on RSA algorithm;
SHA-1 – secure hash algorithm, developed and used by U.S. government
Skipjack – symmetric key algorithm classification is done in hardware Fortezza, used by U.S. government
Triple-DES – DES encryption three times.
Secure sockets layer (SSL) protocol, the dominant mode for secure browser-server communications. (Image courtesy of MIT OCW)
-Step 1: Download JavaMail , an external library from SUN on http://java.sun.com/products/javamail/downloads/index.html
-Step 2: Import JavaMail by Eclipse
-Step 3: Import some packages form JavaMail in code
import java.util.Properties;
import javax.activation.DataHandler;
import javax.mail.Folder;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.Session;
import javax.mail.Store;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
Properties props = new Properties();
//IMAPS protocol
props.setProperty(“mail.store.protocol”, “imaps”);
//Set host address
props.setProperty(“mail.imaps.host”, imaps.gmail.com);
//Set specified port
props.setProperty(“mail.imaps.port”, “993”);
//Using SSL
props.setProperty(“mail.imaps.socketFactory.class”, “javax.net.ssl.SSLSocketFactory”);props.setProperty(“mail.imaps.socketFactory.fallback”, “false”);
//Setting IMAP session
Session imapSession = Session.getInstance(props);
Store store = imapSession.getStore(“imaps”);
//Connect to server by sending username and password.
//Example mailServer = imap.gmail.com, username = abc, password = abc
store.connect(mailServer, account.username, account.password);
//Get all mails in Inbox Forlder
inbox = store.getFolder(“Inbox”);
inbox.open(Folder.READ_ONLY);
//Return result to array of message
Message[] result = inbox.getMessages();
1 – Simple Mail Transfer Protocol (SMTP) is an Internet Standard for e-mail transmission across Internet Protocol networks. SMTPS – SMTP using SSL
2 – Some sample code to connect and send email on Gmail in java as below:
-Step 1: Still import JavaMail library as Step 1, 2, 3 in IMAP
-Step 2: Create a class Email Authentication as below:
package com.glandore.fda.utils.email;
import javax.mail.Authenticator;
import javax.mail.PasswordAuthentication;public class EmailAuthenticator extends Authenticator {
private EmailAccount account;
public EmailAuthenticator(EmailAccount account) {
super();
this.account = account;
}
//Override getPasswordAuthentication to transfer emailAddress and password to get an authentication.
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(account.emailAddress, account.password);
}
}
// Email account class to contain email information.package com.glandore.fda.utils.email;
public class EmailAccount {
public String urlServer = “gmail.com”;
public String username = “namheo”;
public String password = “xxxxx”;
public String emailAddress;
public EmailAccount(String username, String password, String urlServer) {
this.username = username;
this.password = password;
this.urlServer = urlServer;
this.emailAddress = username + “@” + urlServer;
}}
-Step 3: Create a properties for session:
//Create an authetication email
EmailAuthenticator authenticator = new EmailAuthenticator(account);
//Create a property for smtp sesion
Properties props = new Properties();
//Setting smtps protocol
props.setProperty(“mail.transport.protocol”, “smtps”);
//Setting stmpHost. Example: smtpHost = smtp.gmail.com
props.setProperty(“mail.host”, stmpHost);
//Setting specified port
props.put(“mail.smtp.port”, “465”);
props.put(“mail.smtp.socketFactory.port”, “465”);
//Setting using SSL
props.put(“mail.smtp.socketFactory.class”, “javax.net.ssl.SSLSocketFactory”);
props.put(“mail.smtp.socketFactory.fallback”, “false”);
props.setProperty(“mail.smtp.quitwait”, “false”);
//Enable authentication
props.put(“mail.smtp.auth”, “true”);
//Setting smtp session
smtpSession = Session.getDefaultInstance(props, authenticator);
-Step 4: Create a ByteArrayDataSource class to convert the body of message to bytes.
package com.glandore.fda.utils.email;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import javax.activation.DataSource;
public class ByteArrayDataSource implements DataSource {
private byte[] data;
private String type;
public ByteArrayDataSource(byte[] data, String type) {
super();
this.data = data;
this.type = type;
}
public ByteArrayDataSource(byte[] data) {
super();
this.data = data;
}
public void setType(String type) {
this.type = type;
}
public String getContentType() {
if (type == null)
return “application/octet-stream”;
else
return type;
}
public InputStream getInputStream() throws IOException {
return new ByteArrayInputStream(data);
}
public String getName() {
return “ByteArrayDataSource”;
}
public OutputStream getOutputStream() throws IOException {
throw new IOException(“Not Supported”);
}
}
-Step 5: Send email
//Example: sender=nam.tran.flash@gmail.com recipients = nam.tran@glandoresystems.com
//body= the body of message, subject= the title of message
MimeMessage message = new MimeMessage(smtpSession);
//Create handler data to contain the body of message in bytes using ByteArrayDataSource
DataHandler handler = new DataHandler(new ByteArrayDataSource(body.getBytes(), “text/plain”));
//Put information in message
message.setSender(new InternetAddress(sender));
message.setSubject(subject);
message.setDataHandler(handler);if (recipients.indexOf(‘,’) > 0)
//Check recipients to send many email address at the same time
message.setRecipients(Message.RecipientType.TO, InternetAddress.parse(recipients));
else
message.setRecipient(Message.RecipientType.TO, new InternetAddress(recipients));
//Send email
Transport.send(message);
Please check the source code here http://www.box.net/shared/k2vzvynl7x
Namheo
how can I run this program?
Wonderful! =)
i tried this code, its compiling however its not running on the emulator at all. Can you tell me where I am going wrong or missing something ?
Thanks 🙂
Great example
Just a quick question
So in the sample code in this page, IMAP was used to read an email while STMP was used to send an email. forgive my background limitations, is it always this way? STMP for sending emails and IMAP for retrieving them?
@Himura:
This is not always this way. Actually, Imap (Internet Message Access Protocol) and POP3(Post Office Protocol) are protocols to retrieve messages, from a mailbox. Smtp(Simple Mail Transfer Protocol) is another protocol but this one can only send an email to a server.
Basically, if you want to read an email, you use Imap or Pop3, and to send one you use SMTP.
Others protocols are existing (Exchange by example), but I don’t know much about it.